Instructor Led Training - Online
FULL STACK DEVELOPER
Learn cutting-edge tools and practices to streamline collaboration, automate workflows, and enhance the efficiency of your IT operations
- Flexible Schedules
- Live Training
- Real Time Projects
CubenSquare
Full Stack Developer Training Overview
Full stack developer training covers front-end and back-end development. It includes HTML/CSS, JavaScript, front-end frameworks/libraries, server-side programming languages, databases, RESTful APIs, version control (e.g., Git), DevOps practices, testing, security, and soft skills. Continued learning is essential due to the constantly evolving nature of web development
Embrace continuous learning in the Digital Era
40 Hrs Instructor-Led Training
Doubt resolving sessions
Learning Materials
Assignments and Projects
Flexible Schedule
Job Assistance
Learning full stack development enables you to build entire web applications from start to finish, giving you a comprehensive skill set highly sought after in the industry.
As a full stack developer, you have the versatility to work on both front-end and back-end aspects of projects, making you valuable to companies of all sizes. Additionally, you gain a deeper understanding of how different parts of a web application interact, leading to more efficient problem-solving and collaboration.
Full stack developers are in high demand as they can handle various aspects of web development projects independently or as part of a team. This versatility opens up a wide range of career opportunities in tech companies, startups, and freelance projects.
Yes, full stack development can be a great choice for beginners as it provides a well-rounded education in web development. Starting with basic front-end technologies like HTML/CSS and gradually progressing to back-end development allows beginners to build a solid foundation while gaining exposure to different areas of web development.
Course Content
- HTML
- CSS
- Java Script
- JQuery
- Angular
- React
- Java
- Spring Boot Framework
- MySQL Database
- Git
- DevOps
- AWS Cloud Computing
1. Introduction to HTML
– What is HTML?
– Basic structure of an HTML document.
– Importance and role of HTML in web development.
2. HTML Elements
– Understanding HTML tags.
– Basic elements like <html>, <head>, <title>, <body>.
– Text elements (<p>, <h1>, <h2>, <strong>, <em>).
3. Lists and Links
– Creating ordered and unordered lists (<ul>, <ol>, <li>).
– Adding hyperlinks (<a>).
4. Images and Multimedia
– Embedding images (<img>).
– Embedding videos and audio (<video>, <audio>).
5. Forms and Inputs
– Creating forms (<form>).
– Different types of form inputs (text, password, checkbox, radio, etc.).
– Form submission and handling (<input type=”submit”>, form action).
6. Tables
– Creating tables (<table>, <tr>, <td>).
– Table headers (<th>).
– Table structure and formatting.
7. HTML5 Features
– New HTML5 elements (<canvas>, <audio>, <video>).
– Geolocation and local storage.
Project Work:
– Create a basic personal website with multiple pages.
– Build a form for collecting user data.
1. Introduction to CSS
– What is CSS?
– Why CSS is important?
– Basic syntax and structure.
2. Selectors and Properties
– Understanding selectors (element, class, ID).
– Basic CSS properties (color, font, background, etc.).
3. Box Model
– Understanding the box model (margin, border, padding).
– Box-sizing property.
4. Layouts
– Introduction to layout techniques (floats, flexbox, grid).
– Responsive design principles.
5. Advanced Selectors and Pseudo-classes
– Combinators and attribute selectors.
– Pseudo-classes (:hover, :active, :focus).
6. Positioning and Display
– Positioning elements (static, relative, absolute, fixed).
– Display property (block, inline, inline-block).
7. Typography and Web Fonts
– Working with text properties (font-size, font-family, line-height).
– Importing and using web fonts.
8. Transitions and Animations
– Transition effects (hover effects, color changes).
– Keyframe animations.
9. Advanced Layout Techniques
– Advanced flexbox and grid techniques.
– Multi-column layouts.
Project Work:
– Build a simple static website using HTML and CSS.
1. Introduction to JavaScript
– What is JavaScript?
– Brief history and importance in web development.
2. Basic Syntax and Data Types
– Variables (var, let, const).
– Data types (strings, numbers, booleans).
– Basic operators (+, -, *, /).
3. Control Flow
– Conditional statements (if, else, else if).
– Comparison operators (==, ===, !=, !==).
– Logical operators (&&, ||, !).
4. Loops
– for loops.
– while loops.
– Loop control statements (break, continue).
5. Functions
– Declaring and invoking functions.
– Function parameters and return values.
– Function expressions and arrow functions.
6. Arrays and Objects
– Creating and manipulating arrays.
– Accessing array elements.
– Working with object literals and properties.
7. DOM Manipulation
– Introduction to the Document Object Model (DOM).
– Selecting and manipulating DOM elements.
– Handling events (addEventListener).
8. Error Handling
– Introduction to exceptions and error handling.
– try, catch, finally blocks.
1. Introduction to jQuery
– What is jQuery?
– Why use jQuery?
– Basics of DOM manipulation.
2. Setting Up jQuery
– Downloading and linking jQuery library.
– Using jQuery via Content Delivery Network (CDN).
3. Selectors and Filters
– Selecting elements by ID, class, tag name, etc.
– Filtering elements based on specific criteria.
4. DOM Manipulation
– Modifying HTML content (html(), text()).
– Manipulating CSS properties (css()).
– Adding/removing classes (addClass(), removeClass()).
5. Event Handling
– Attaching event handlers (click(), hover(), submit()).
– Event delegation and propagation.
6. Effects and Animations
– Basic effects (show(), hide(), toggle()).
– Animations (animate(), fadeIn(), fadeOut()).
7. Traversing the DOM
– Navigating the DOM hierarchy (parent(), children(), siblings()).
– Filtering and searching elements (filter(), find()).
Beginner Level:
1. Introduction to Angular
– What is Angular?
– Angular architecture and features.
– Setting up a development environment.
2. Basic Concepts
– Components and templates.
– Data binding (interpolation, property binding, event binding).
– Directives (structural and attribute directives).
3. Modules and Dependency Injection
– Understanding Angular modules.
– Dependency injection and its importance.
4. Routing
– Setting up and configuring Angular Router.
– Routing parameters and navigation.
5. Services and HTTP Client
– Creating and using Angular services.
– Making HTTP requests with HttpClient module.
– Handling responses and errors.
6. Forms
– Template-driven forms.
– Reactive forms with FormBuilder and FormGroup.
7. Component Interaction
– Input and output properties.
– Component communication using @Input and @Output decorators.
– Parent-child and sibling component communication.
8. Pipes and Directives
– Using built-in pipes (e.g., date, uppercase, lowercase).
– Creating custom pipes.
– Creating custom directives.
Project Work:
– Build a simple CRUD application with Angular.
1. Introduction to React
– What is React?
– Virtual DOM concept.
– React’s component-based architecture.
2. Setting Up a React Environment
– Installing Node.js and npm.
– Creating a new React project with Create React App.
– Exploring the project structure.
3. JSX and Components
– Introduction to JSX syntax.
– Creating functional and class components.
– Rendering components in the DOM.
4. Props and State
– Understanding props and state.
– Passing props to components.
– Managing state with setState().
5. Handling Events
– Event handling in React.
– Binding event handlers.
– Passing arguments to event handlers.
6. Lifecycle Methods
– Understanding component lifecycle.
– ComponentDidMount, ComponentDidUpdate, and ComponentWillUnmount.
7. React Hooks
– Introduction to hooks (useState, useEffect).
– Using hooks to manage state and side effects.
8. Lists and Keys
– Rendering lists with map().
– Understanding the importance of keys.
Project Work:
– Build a simple Todo list application.
– Create a weather app that fetches data from an API
1. Introduction to Java
– What is Java?
– Setting up Java Development Environment (JDK, IDEs).
2. Basic Syntax and Data Types
– Java syntax rules and conventions.
– Primitive data types (int, double, boolean, etc.).
– Variables and constants.
3. Control Flow
– Conditional statements (if, else-if, switch).
– Looping constructs (for, while, do-while).
4. Arrays
– Declaring and initializing arrays.
– Array manipulation (accessing elements, iterating, sorting).
5. Object-Oriented Programming (OOP)
– Introduction to OOP concepts (classes, objects, inheritance, polymorphism, encapsulation).
– Defining classes and objects in Java.
6. Methods and Functions
– Declaring and invoking methods.
– Method overloading and overriding.
– Passing arguments and returning values.
7. Exception Handling
– Handling exceptions using try-catch blocks.
– Throwing custom exceptions.
8. File Handling
– Reading from and writing to files.
– Working with file streams and buffers.
9. Generics
– Understanding generics and type parameters.
– Generic classes and methods.
10. Java Database Connectivity (JDBC)
– Connecting to databases using JDBC.
– Executing SQL queries from Java programs.
Spring Framework Fundamentals:
1. Introduction to Spring Framework
– Overview of Spring framework and its modules.
– Dependency Injection and Inversion of Control (IoC) principles.
2. Spring Core
– Bean configuration using XML and annotations.
– Spring Bean lifecycle.
3. Spring MVC
– Introduction to Model-View-Controller architecture.
– Creating controllers, handling requests, and returning responses.
4. Spring Data Access
– Integrating Spring with JDBC for database access.
– Using Spring Data JPA for ORM.
5. Introduction to Spring Boot
– Overview and advantages of Spring Boot.
– Setting up a Spring Boot project.
6. Spring Boot Starter
– Understanding starter dependencies.
– Auto-configuration and customization.
7. RESTful Web Services with Spring Boot
– Creating RESTful APIs.
– Handling HTTP methods (GET, POST, PUT, DELETE).
8. Spring Boot Data
– Working with databases using Spring Boot Data.
– Implementing CRUD operations.
9. Security with Spring Boot
– Implementing authentication and authorization.
– Securing endpoints.
1. Introduction to Databases
– What is a database?
– Types of databases (SQL vs. NoSQL).
– Introduction to MySQL.
2. Basic SQL Syntax
– Understanding SQL statements (SELECT, INSERT, UPDATE, DELETE).
– Creating and dropping databases and tables.
– Data types in MySQL.
3. Querying Data
– SELECT statement and its clauses (FROM, WHERE, ORDER BY, LIMIT).
– Filtering and sorting data.
– Using aggregate functions (COUNT, SUM, AVG, MAX, MIN).
4. Modifying Data
– INSERT statement for adding data.
– UPDATE statement for modifying data.
– DELETE statement for removing data.
5. Joins and Relationships
– Understanding different types of joins (INNER JOIN, LEFT JOIN, RIGHT JOIN, FULL JOIN).
– Establishing relationships between tables (primary key, foreign key).
6. Subqueries and Derived Tables
– Writing subqueries within SELECT, WHERE, and FROM clauses.
– Using derived tables in SQL queries.
7. Indexes and Optimization
– Understanding indexes and their types (B-tree, Hash, etc.).
– Creating and managing indexes.
– Query optimization techniques.
8. Views and Stored Procedures
– Creating and managing views.
– Writing and executing stored procedures.
9. Transactions and Concurrency
– Understanding transactions and their properties (ACID).
– Managing transactions in MySQL.
– Handling concurrency issues.
10. User Management and Security
– Creating and managing user accounts.
– Granting and revoking privileges.
– Best practices for securing MySQL databases.
11. Backup and Recovery
– Performing database backups (logical vs. physical).
– Implementing recovery strategies.
12. Advanced SQL Techniques
– Window functions.
– Common table expressions (CTEs).
– Recursive queries.
1. Introduction to Version Control
– Understanding version control systems.
– Importance of version control in software development.
2. Installing Git
3. Basic Git Concepts
– Repository (repo) and working directory.
– Git commands: init, add, commit, status, log.
4. Working with Remote Repositories
– Cloning a repository from a remote server (git clone).
– Adding remote repositories (git remote).
– Pushing changes to a remote repository (git push).
5. Branching and Merging
– Creating branches (git branch).
– Switching branches (git checkout).
– Merging branches (git merge).
6. Collaborating with Others
– Fetching changes from a remote repository (git fetch).
– Pulling changes from a remote repository (git pull).
– Resolving merge conflicts.
7. Git Workflow
– Understanding different Git workflows (e.g., Centralized, Feature Branch, Gitflow).
– Choosing an appropriate workflow for your project.
8. Tagging and Releases
– Creating tags for releases or specific commits (git tag).
– Managing releases in Git.
Introduction to DevOps:
1. Understanding DevOps
– Definition and principles of DevOps.
– Importance of DevOps in modern software development.
– DevOps culture and practices.
2. DevOps Lifecycle
– Overview of DevOps lifecycle stages (Plan, Develop, Build, Test, Deploy, Operate, Monitor).
– Continuous Integration (CI) and Continuous Delivery (CD) pipeline.
Version Control Systems:
3. Introduction to Version Control
– Understanding version control systems.
– Importance of version control in DevOps.
– Git basics and best practices.
4. Version Control Tools
– Deep dive into Git features and commands.
– Using Git for collaborative development.
Continuous Integration and Continuous Delivery (CI/CD):
5. CI/CD Concepts
– Definition and benefits of CI/CD.
– CI/CD pipeline components.
– Continuous Integration with tools like Jenkins, GitLab CI/CD, or Travis CI.
6. Automated Testing
– Introduction to automated testing (unit tests, integration tests, end-to-end tests).
– Integrating automated tests into CI/CD pipelines.
Infrastructure as Code (IaC):
7. Introduction to Infrastructure as Code
– Definition and benefits of IaC.
– Infrastructure provisioning tools (e.g., Terraform, AWS CloudFormation, Azure Resource Manager).
8. Configuration Management
– Introduction to configuration management tools (e.g., Ansible, Puppet, Chef).
– Managing infrastructure configurations declaratively.
Containerization and Orchestration:
9. Containerization Basics
– Introduction to containers (Docker).
– Containerization benefits and use cases.
10. Container Orchestration
– Introduction to container orchestration platforms (Kubernetes, Docker Swarm).
– Deploying and managing containerized applications at scale.
Monitoring and Logging:
11. Monitoring Tools
– Introduction to monitoring tools (Prometheus, Grafana, ELK stack).
– Monitoring infrastructure and application metrics.
12. Log Management
– Introduction to log management tools (ELK stack, Splunk).
– Collecting, analyzing, and visualizing log data.
1. Introduction to Cloud Computing
– What is cloud computing?
– Advantages of cloud computing.
– Overview of AWS cloud services.
2. Getting Started with AWS
– Creating an AWS account.
– Navigating the AWS Management Console.
– Understanding AWS regions and availability zones.
3. Amazon EC2 (Elastic Compute Cloud)
– Launching and configuring EC2 instances.
– Connecting to EC2 instances.
– Security groups and key pairs.
4. Amazon S3 (Simple Storage Service)
– Creating S3 buckets.
– Uploading and managing objects in S3.
– Setting up permissions and access control.
5. AWS Identity and Access Management (IAM)
– Understanding IAM users, groups, and roles.
– Managing IAM policies.
– Implementing security best practices.
6. Amazon RDS (Relational Database Service)
– Deploying and managing RDS instances.
– Configuring database backups and snapshots.
– Monitoring RDS performance.
7. Amazon VPC (Virtual Private Cloud)
– Creating VPCs, subnets, and route tables.
– Configuring security groups and network ACLs.
– Connecting VPCs using VPN and Direct Connect.
8. AWS Lambda
– Understanding serverless computing.
– Creating and deploying Lambda functions.
– Integrating Lambda with other AWS services.
CubenSquare
Students from Across
the Globe

CubenSquare
Peer Learning
Our commitment to fostering a collaborative environment ensures that every member of our community contributes to and benefits from the wealth of knowledge within our diverse network.
Networking
A community is formed were all members interact and help each other – during and after the training along with Mentor Guidance
- Collaborative Workspaces
- Knowledge Sharing Sessions
- Troubleshooting
- Continuous Learning Culture
CubenSquare
Certification
This Course involves – Training and Certification from CubenSquare
- Hands on Training
- Real Time Projects
- Assisgnments
- Course Completion Certificate
- Job Assistance
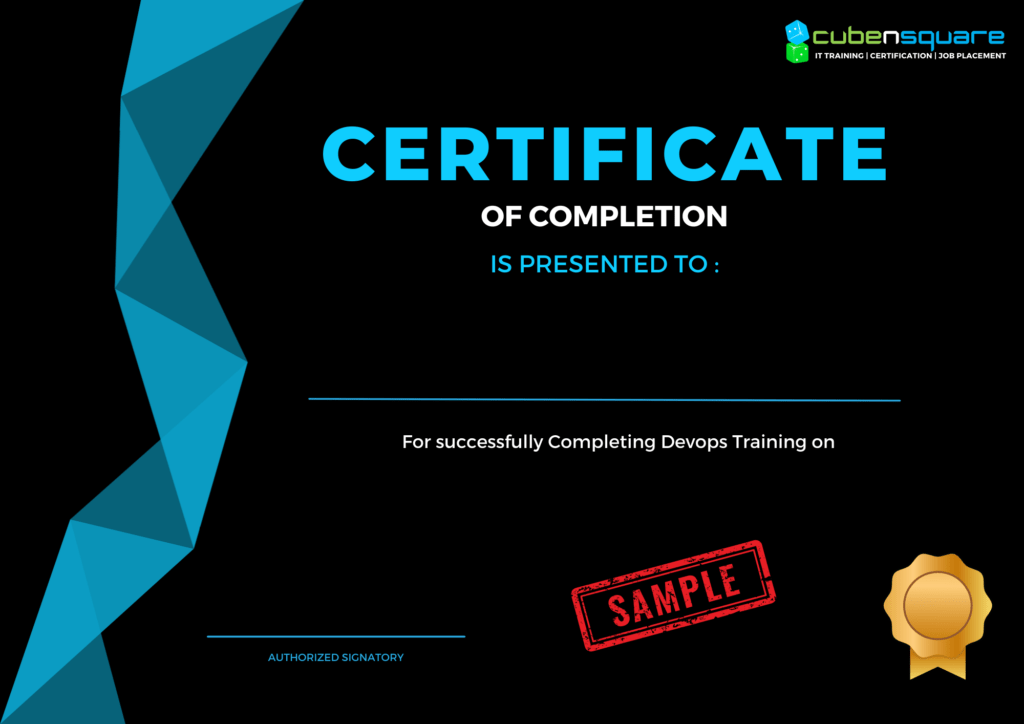
CubenSquare
Our Alumni Works At







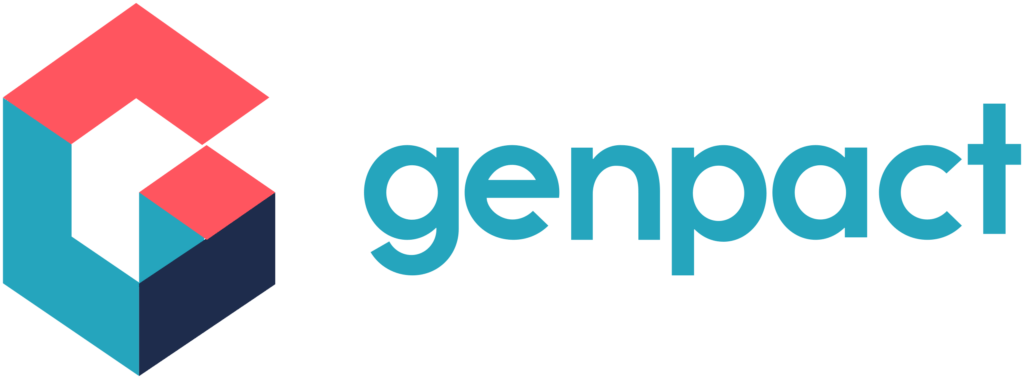



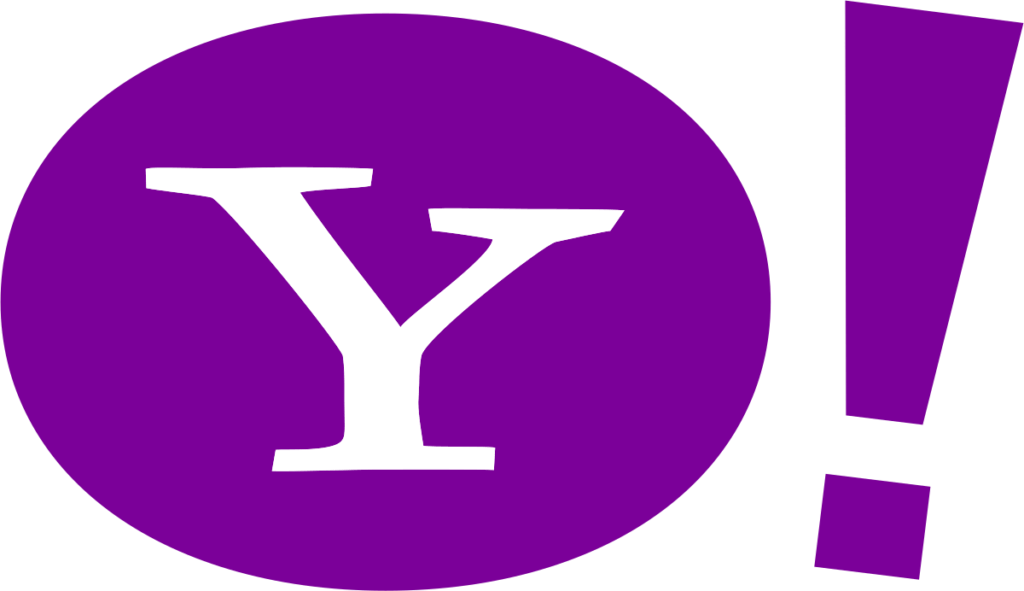











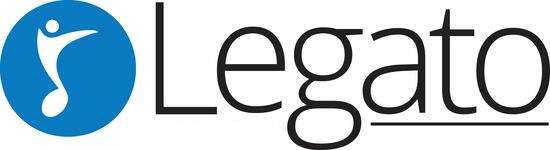




Career Roadmap
CubenSquare
Testimony
ExcellentBased on 299 reviews![]()
DIVYA GANESH2023-12-15Best place to learn devops 😃😃😃😃😃😃for beginners Gomz best trainer 😇😇to assist us to learn all the concepts .
Prabhu_devops Prabhumdu2023-12-15I have completed Devops here… For fresher and experienced peoples this will be the turning point in our life… Literally saying… Before class starts we will have many things in your mind… But once class started… Goms will not leave your mind to think apart from his voice…until he say’s “Thank you guys will see you in next class” 100% worth to attend his class… Don’t think about the course… surely he will not leave you without filling in your mind… Classic and real life examples.. 24/7 reachable..and will give more confidence to attend interviews… Thanks Cubensquare and Goms…
revathi k2023-12-14Hi,I am revathi recently i have completed Devops & Openshift course in cubensquare.I Just want to pass on some honest feedback of my tutor&mentor(Goms) and the institution.He concentrate on the practical knowledge rather than therory.Goms willing to help any student at anytime.He has a passion for his students to succeed in and beyond the classroom.The course is without a doubt one of the best investments I have made in myself. Goms explain the topics with real time examples which we never cant forget.They offers the various trainings and internship for students and experienced.Cubensquare is the result of hard work, dedication, and a lot of sleepless nights. Congratulations on this amazing achievement.I Wish to be part of your team :)
ranjith vinayagam2023-12-13For the past one year I am learning various courses from cubensquare terraform,red hat and now dev ops. Goms sir’s teaching methodology was unique and he makes us understand the concepts really well.I am happy that I choose this institute to learn dev ops.Every single penny i paid for this course is really worth it
Ajmal Sheriff2023-12-13Good Institute to learn Devops Course. Trainer Goms helped us to understand each and every concepts clearly. All classes are practical oriented with real time scenarios/examples which makes everyone easy to learn. We will also get a good opportunity to do a real time Project.
Kanaga Valli2023-12-13I was considering taking a course but wasn't sure what to choose and was unsure whether I could accomplish it. Goms sir is the one who gave me confidence and helped me become an expert in both openshift and DevOps. I begin both concepts with no prior understanding of openshift and DevOps. Now I understand what to do with openshift and DevOps. He clarified all of my clarifications. I've never seen such a dedicated teacher in my life. Thank you once more, Gom Sir
Zakirhussain Noorulla2023-12-13The Best place to 1.Enhance your carrier 2.Change your Non-IT domain to IT domain 3.Get your dream job with good hike. I have done my devOps course and Redhat openshift certification recently in CubenSquare. Pros : 1.Very skilled trainers for each technology. Specially our trainer GOMS, the way he teach each topic with correlated realtime examples (live projects) in layman terms makes better understanding and register in our mind easily. 2. They provide very clear live running notes for each class with recordings, with all presentation, nowhere institute will provide this .So after years also we can easily refer incase of doubts. 3. I personally like, the classes are much more practical sessions rather just showing PPT and boring. 4. They follow perfect class timings, No last minute changes. At rare case if any changes we get to informed before itself. 5. Both weekdays/weekend and online/offline available. 6.Very reasonable price for each course, mainly they have easy instalment option which is helpful for most of students. 7. Good placement opportunities as being recruitment partners with TATA and other organisation. 8. All over best place to make your carrier better with all needed stuffs in technologies . Cons: Honestly No🙅
Selvaganapathy2023-12-13CubenSquare is the Best place to learn Devops , Redhat Openshift, Ansible and other offered cources in an effective way with real time examples and gain knowledge with live scenarios & problem solutions. trainer Mr.Goms is the best person to teach these technologies from scratch & making the people expert in their domain. Also they offer with technology experts working on real time is very much helpful to know & feel the work and present in the interview and they guide and support until we get a job. I appreciates CubenSquare teams and support they provided during my learning path. Thank you Cubensquare!!!
DHANUSH Danu2023-12-13I really enjoyed this class and the format it was presented in. For me, I learn and retain much more through an online class. I retain more information. I found it relaxing to be able to turn the work on the assignments and test at my leisure and when I had the time. To be honest, there is nothing that I disliked about the course. Special thanks to Goms for guiding me. Thank you once again.
Request a Quote
Ready to Upskill? Contact us now!
Learn More From
Frequently Asked Questions
All classes are available – Online and Offline
All classes are Live led by an instructor
Yes for all students